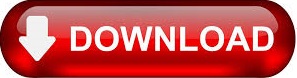
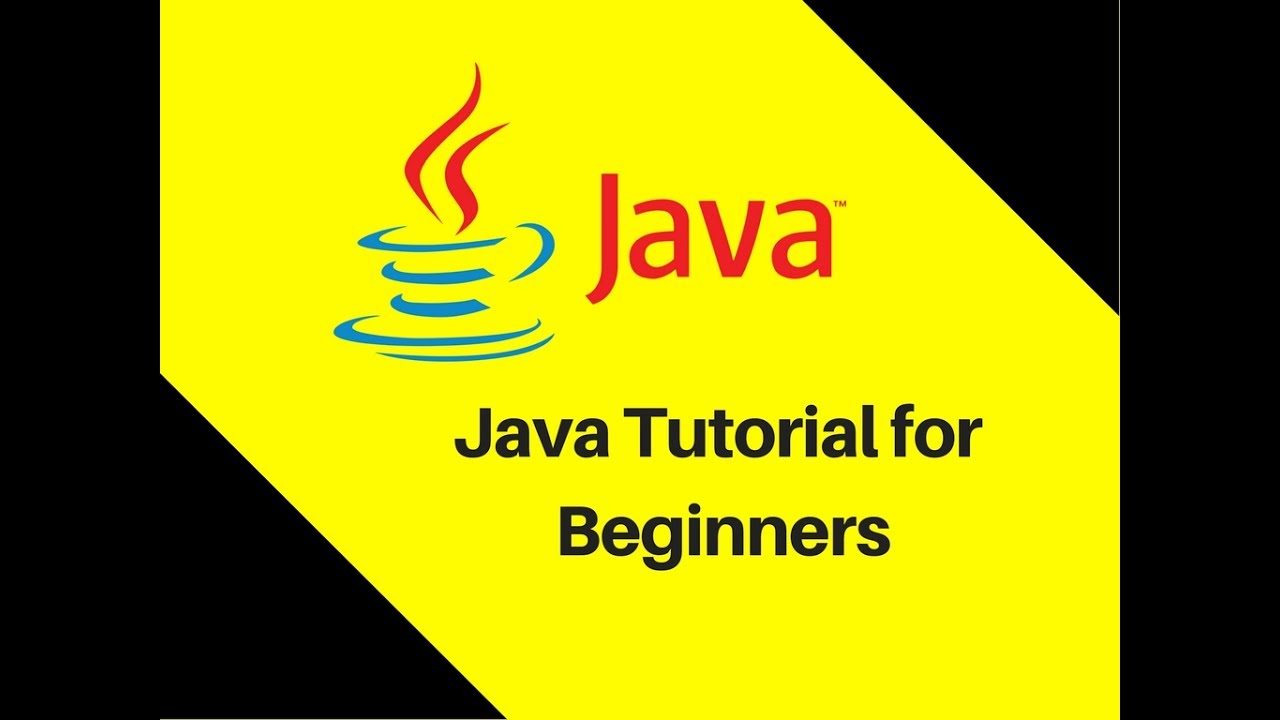
#Java reflection tutorial for beginners code#
USING OBJECTS Here, code in one class creates an instance of another class and does something with it … Fruit plum=new Fruit() int cals cals = plum. PUBLIC/PRIVATE Methods/data may be declared public or private meaning they may or may not be accessed by code in other classes … Good practice: keep data private keep most methods private well-defined interface between classes - helps to eliminate errors STATEMENTS & BLOCKS A simple statement is a command terminated by a semi-colon: name = “Fred” A block is a compound statement enclosed in curly brackets: ĪSSIGNMENT All Java assignments are right associative int a = 1, b = 2, c = 5 a = b = c ( “ a= “ + a + “b= “ + b + “c= “ + c) What is the value of a, b & c Done right to left: a = (b = c) īASIC MATHEMATICAL OPERATORS * / % + - are the mathematical operators * / % have a higher precedence than + or - double myVal = a + b % d – c * d / b Is the same as: double myVal = (a + (b % d)) – ((c * d) / b) 1.2 is a double value accurate to 15 decimal places. INITIALISATION If no value is assigned prior to use, then the compiler will give an error Java sets primitive variables to zero or false in the case of a boolean variable All object references are initially set to null An array of anything is an object Set to null on declaration Elements to zero false or null on creation ĭECLARATIONS int index = 1.2 // compiler error boolean retOk = 1 // compiler error double fiveFourths = 5 / 4 // no error! float ratio = 5.8f // correct double fiveFourths = 5.0 / 4.0 // correct 1.2f is a float value accurate to 7 decimal places. Declaring primitive variables: float initVal int retVal, index = 2 double gamma = 1.2, brightness boolean valueOk = false This means that you don’t use the new operator to create a primitive variable.
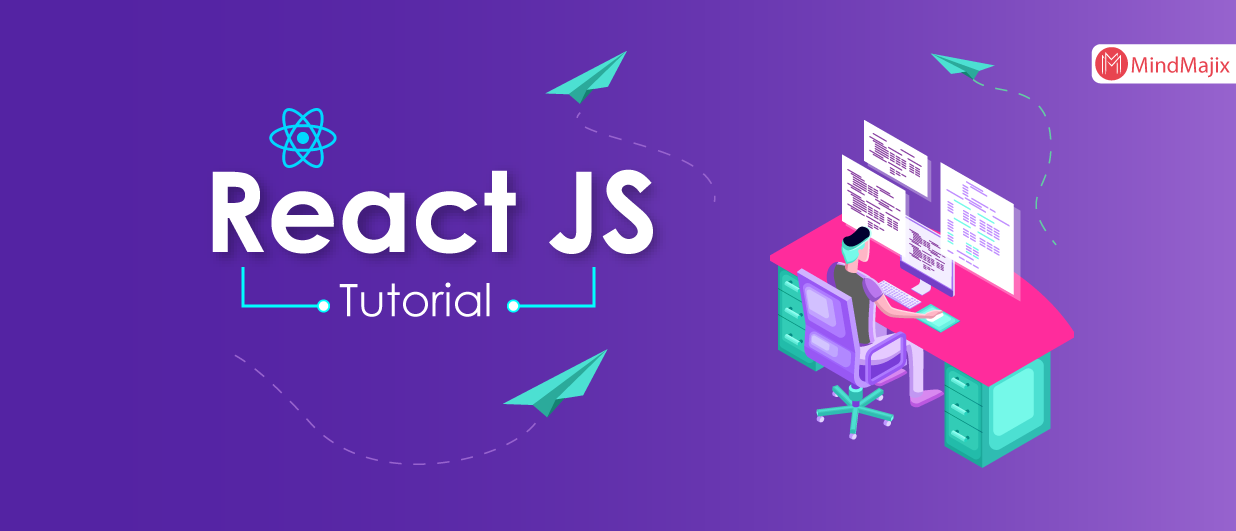
These basic (or primitive) types are the only types that are not objects (due to performance issues).
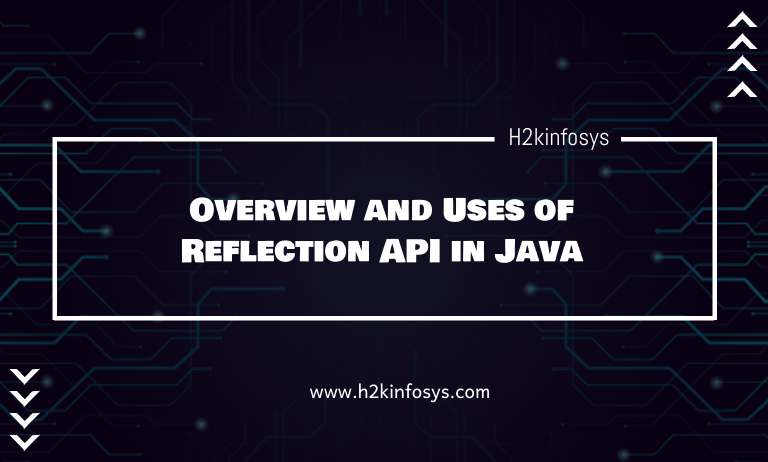
PRIMITIVE TYPES AND VARIABLES boolean, char, byte, short, int, long, float, double etc.
#Java reflection tutorial for beginners portable#
JAVA ADVANTAGES Portable - Write Once, Run Anywhere Security has been well thought through Robust memory management Designed for network programming Multi-threaded (multiple simultaneous tasks) Dynamic & extensible (loads of libraries) Classes stored in separate files Loaded only when needed OBJECT-ORIENTED Java supports OOD Polymorphism Inheritance Encapsulation Java programs contain nothing but definitions and instantiations of classes Everything is encapsulated in a class! JAVA - SECURITY Pointer denial - reduces chances of virulent programs corrupting host, Applets even more restricted - May not run local executables, Read or write to local file system, Communicate with any server other than the originating server. HOW IT WORKS…! Java is independent only for one reason: Only depends on the Java Virtual Machine (JVM), code is compiled to bytecode, which is interpreted by the resident JVM, JIT (just in time) compilers attempt to increase speed. HOW IT WORKS…! Compile-time Environment Compile-time Environment Java Bytecodes move locally or through network Java Source (.java) Java Compiler Java Bytecode (.class ) Runtime System Class Loader Bytecode Verifier Java Class Libraries Operating System Hardware Java Virtual machine Java Interpreter Just in Time Compiler JAVA - GENERAL Java has some interesting features: automatic type checking, automatic garbage collection, simplifies pointers no directly accessible pointer to memory, simplified network access, multi-threading! JAVA - GENERAL Java is: platform independent programming language similar to C++ in syntax similar to Smalltalk in mental paradigm Pros: also ubiquitous to net Cons: interpreted, and still under development (moving target)
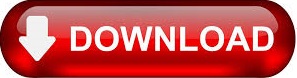